Hi guys, hope you all doing well. I had some free time and decided to share some ideas on how to approach resource recollection with pixelbots. If you didn't read my post on how to detect the dofus client, check pixel coordinates and colors, detect fights and autofight, you can always take a look on this. Lets start.
1. Collect resources
Approach 1: Click although the resource has not reappeared
This is the easiest way of collecting resources. The idea is to click on the resource location even if someone has collected it and the resource has not reappeared. This approach allows to save some lines of code. At first glance seems like a huge waste of time, but after dozens of hours of testing and collecting resources, I think this approach is efficient enough to take it into consideration since only consumes 3 to 5 seconds per resource spot. The algorithm would be something like this:
- Click on a resource spot coordinates
- Wait 3 to 5 seconds.
- Check if a fight has started (a resource protector has appeared) --> fight detector
- If yes --> fight
- If no --> continue recollecting
- Click on another resource spot on the same map
Once the map is collected, change map and continue the recollection.
Approach 2: Only click if the resource has reappered and is collectable.
This is the difficult approach but the most efficent one. You need to know what chroma pallet a specific resource uses, and search for it on the screen. If any resource is collectable, you can continue your route without wasting time clicking on empty resource spots. The way I found to detect resources is this one:
- Imagine I want to collect some chestnut trees. Then I need to search for the colors of that tree. I always try to search for the less common color of the resource. For example chestnut trees usually have this small chestnut in the treetop.
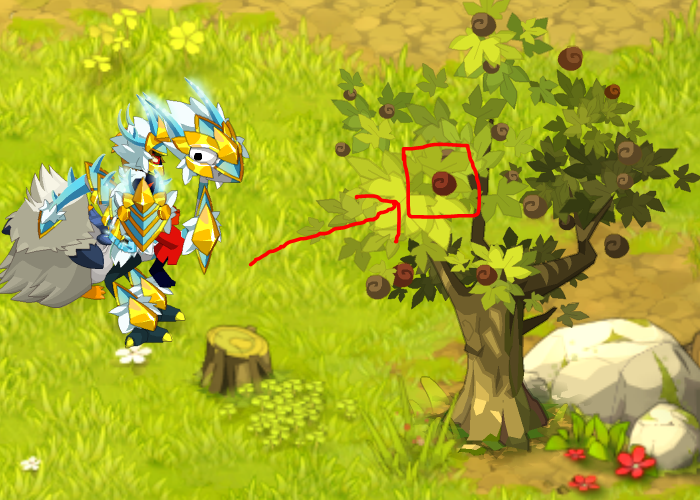
- You need to know that even if two resources are of the same type, their colors might not be always the same, they follow a pallet, and they vary some hex values. Maybe one chestnut color (in hexadecimal) is AB3D26A1 and another one in the same map is AB3D26A2. So it's not possible to search for a specific hex value, but instead you need to search using a regex pattern. Pls dont try to check colors directly on the Dofus client because the white aura that appears around the resource in fact alters (slightly) all the colours of the resource.
- Take a screenshot and then check the colour of the desired area of your resource. For example lets take a look on some chestnuts, and compare their colors:![img]()
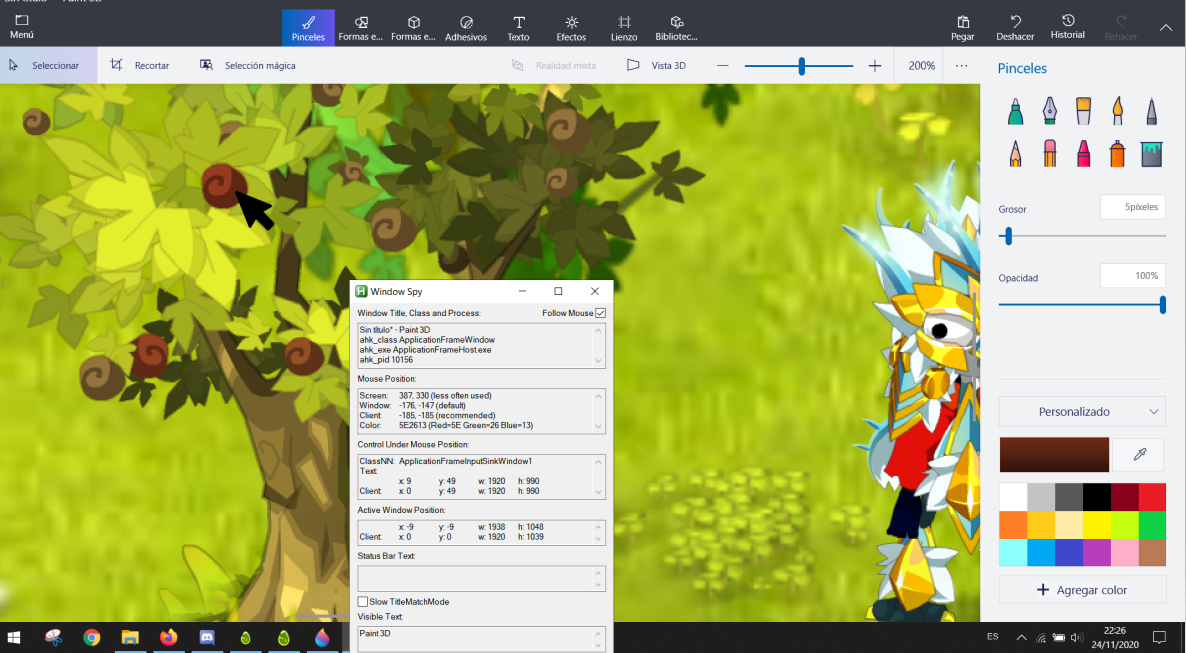
As you can see, we are checking the colours of the same type of tree, on the same map, BUT they vary slightly. The first one is 5B2413 and the second is 5E2613. I don't know exactly why this happens, if it's intended by Ankama to avoid pixel bots or what but the fact is: you need to search colour resources using regex, and not specific hex values.
So, how we do this? Take another look on those hex values, they share the same number structure: 5X2X1X, so basically thats your RegEx.
checkColor(color){
c := color
if RegExMatch(c, "^0x6.2.1.$")=1 or RegExMatch(c, "^0x5.2.1.$")=1
return 0
else
return 1
}
You can see I also compare it with 6x2x1x and thats because thats another hex colour pattern for chestnuts. So, thats all for resource detection.
2. Routes
The first step when coding a route is planning the route. In order to do that, I think one solution is to decide what resource/resources do you want and then check dofus maps online.
Lets say we want some chestnuts, we check the map and we plan our route. This is a example of a mini route of chestnuts from zaap Scaraleafs. Its only an example, is not efficient probably and of course is a small route, which has many problems related to the Ankama Antibot IA as we will discuss later.
![img]()
So, now you have your route planned, and you know how to collect resources. Also you can autofight resource protectors if you saw my last post. So whats next? Well we need to discuss how to actually move between maps. To change map, simply click on the blue areas, any clicks near that areas will make your character move and change to the adjacent map on that direction.
![img]()
But now, how we know we have changed maps and we can continue running the script?
The approach I follow is map has changed. It sounds weird but I think its efficient. First you need to make sure the lateral bars of your Dofus Client shows the adjacent maps. Then the only task is run a Loop that waits forever until it detects the map has changed, then the loop breaks, and the next resources can be collected. How to do this?
Select some random pixels from areas 1, 2, 3 and/or 4, and check that they are all colour black. I recommend to at least check 3 different areas. Whenever you change maps, those 4 areas turn black until the next map is loaded and is displayed. So when you have finished collecting one map, click to change maps, and then run a loop that checks those areas.
MoveRight(){
Click, 1650, 450
Loop{
PixelGetColor, color, 100, 100
PixelGetColor, color2, 350, 350
PixelGetColor, color3, 700, 700
} Until (color = 0x000000 and color2 = 0x000000 and color3 = 0x000000)
Sleep 1500
return
}
Now, I will give you some tips I have learned after all this time.
- To make some routes on an infinite loop... make an infinite loop hehe and put inside your different routes.
- To use zaaps, go to your Haven Bag (Havre-Sac (?) ) by pressing H then click on your zaap and type the desired zaap with Send nameofthezaap then send Enter key. The move to the initial map of your route and start collecting.
- After you change map, always Send Shitf key down, that will make sure that your character remembers and collects all the resources even if you are attacked, or some lag appears, and most important, to make sure you dont attack enemies! This is so important in areas like the Abyss of Sufokia. If the Shift key is down, even if you click on a monster moob, you will not enter in combat, just the moob info coordinates will appear on the chat.
3. AntiBot Ankama IA + Anti Player Bot Reports
Well this is an easy approach. Since Ankama (or at least I have read that) checks for repetitive tasks, actions or routes to autoban bots (I don't know if its real, but just in case xD ) always make sure you run large routes that covers different areas. For example Otomai, then Frigost, then Amakna, then Sufokia then whatever and then you can repeat the loop. I have checked my routes, and my character usually takes like 50 minutes to finish one loop of the route. That means even if a player/mod is looking for bots, he will have to wait about two hours to see you go through one specific map again. Nobody does that, so basically its antiban 99,99%. I have been testing my scripts for 8 hours daily (while I sleep), every day for 2 weeks, and never had problems. (Btw all the resources I got, about 40 million stimated kamas, I never sold them or craft them because its not fair with the other players. I just stored it all on one of my house chests because I just wanted to test how much stuff could this type of bot collect and said to my friends to take as much as they wanted).
4. Auto Pods + Auto Bank
Put the remaning pods on your character bar, and every time you finish a collecting loop, check if it has reached the limit (the green bar is on the right side).
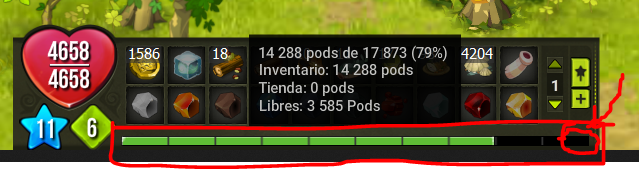
Then trigger a method to go to Bonta (go to your havre-sac by pressing H, click on the zaap, write Bonta by using the Send Bonta command then Send Enter key, click on the zaapi, click on the right menu, click on the textbox, Send bank, Send Enter, click on the bank door, click on the owl to speak with him, click on open my account..) well I think you understand how to do it. Click on your resource interface, click on the arrow, click on "transfer the visible items", click on the X to close the bank interface and you are ready to continue your collecting loop. If you dont want to use the bank, and you have a house, apply the same logic but going to your house and use your chest.
Video demo with the start of a route + auto detect resources + auto collect: Video
Example of script (1000 lines of code with 4 complete routes + pods + house) using the Approach 1: Script
Remember that my scripts are for 1920*1080 with 125% zoom and if a fight is detected you need the scripts I shared in my first post if you want to autofight.
![img]()
Happy Farming!
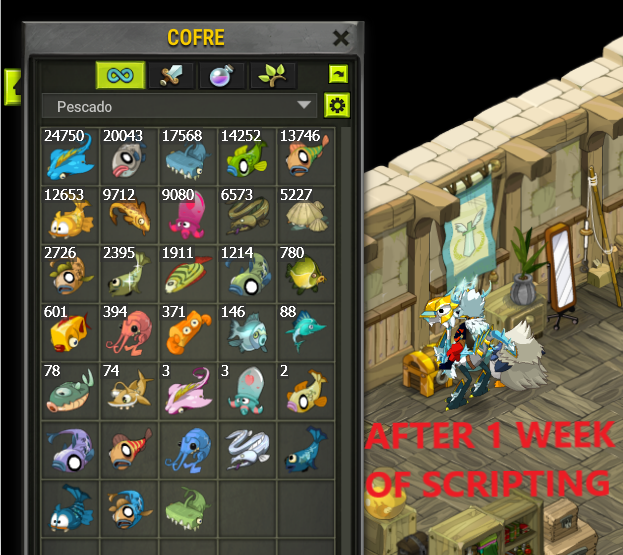
![img]()
AutoEnclos breed + generations, auto Treasure Hunts...and more, much more... coming soon!